LeetCode-101. Symmetric Tree
難度: easy
Given the
root
of a binary tree, check whether it is a mirror of itself (i.e., symmetric around its center).
Example 1:
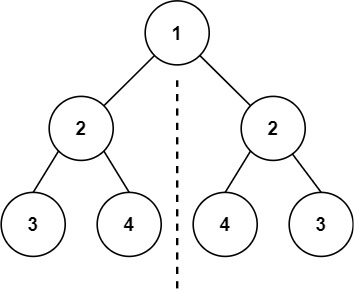
root = [1,2,2,3,4,4,3]
Output: true
Example 2:
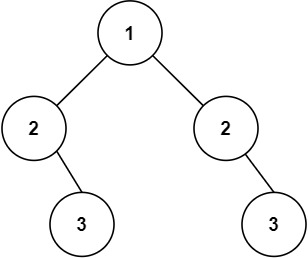
root = [1,2,2,null,3,null,3]
Output: false
Constraints:
- The number of nodes in the tree is in the range
[1, 1000]
. -100 <= Node.val <= 100
解題流程
- 先看懂題目想描述甚麼情境
- 開始想像程式運作該經過哪些流程
- 將經過的流程所需的判斷跟細節列出
- 實作列出的流程
先看懂題目想描述甚麼情境
開始想像程式運作該經過哪些流程
將經過的流程所需的判斷跟細節列出
實作列出的流程
typescript
分而治之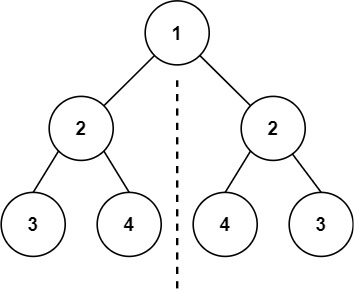
/**
* Definition for a binary tree node.
* class TreeNode {
* val: number
* left: TreeNode | null
* right: TreeNode | null
* constructor(val?: number, left?: TreeNode | null, right?: TreeNode | null) {
* this.val = (val===undefined ? 0 : val)
* this.left = (left===undefined ? null : left)
* this.right = (right===undefined ? null : right)
* }
* }
*/
function isSymmetric(root: TreeNode | null): boolean {
if(!root) return true;
function isMirror(leftNode: TreeNode | null, rightNode: TreeNode | null) {
if(!leftNode && !rightNode) {
return true;
}
if(
leftNode?.val === rightNode?.val &&
isMirror(leftNode.right, rightNode.left) &&
isMirror(leftNode.left, rightNode.right)
) {
return true;
}
return false;
}
return isMirror(root.left, root.right);
};
感謝閱讀,如果有錯誤或是講得不清楚的部分,再請留言指教